In the previous post, we prepared the contract and wrote the test codes. In this post, we will make the contract interact with UI and call the functions defined in the contract. If you haven’t read the previous post, you can reach it from the following link.
Compiling the Contract and Publishing it in the Network
We need first to publish the contract in the blockchain network. We will use the local blockchain network, Ganache, as we did in the previous post. When we run the command below, there will be a deployment to Ganache according to the network definition in the file “truffle-config.js”. We did that definition in the previous post.
truffle migrate --reset
Contracts are sent to the blockchain network in bytecode after they have been compiled. Therefore, we need an interface in order to interact with contracts. This interface is called ABI. When a contract is compiled, json output is created. This json output has ABI. We can see this json under the folder “build/contracts” when we run the above code. Besides the interface, we will also need the contract address in the blockchain network. We can see the contract address in the console. Let’s take a note of this.
Installing MetaMask and Configuring Ganache Network in MetaMask
We saw in the previous post that Ganache provided us with 10 accounts with balance of 100ETH when we were writing test. We called and tested the contract functions by using these accounts. Now that we are going to develop a UI application, we need a wallet that can be used in the browser. This wallet is MetaMask. MetaMask allows us to interact with the blockchain network. It signs our transactions and sends them to the network. You can install it to your browser as an extension from the following link.
We will need to create an account after the installation. We will carry out our tests in Ganache. So we need to connect MetaMask to Ganache. We go to the Custom RPC from the networks section and add the information there as below. Don’t forget to ensure that Ganache is running.
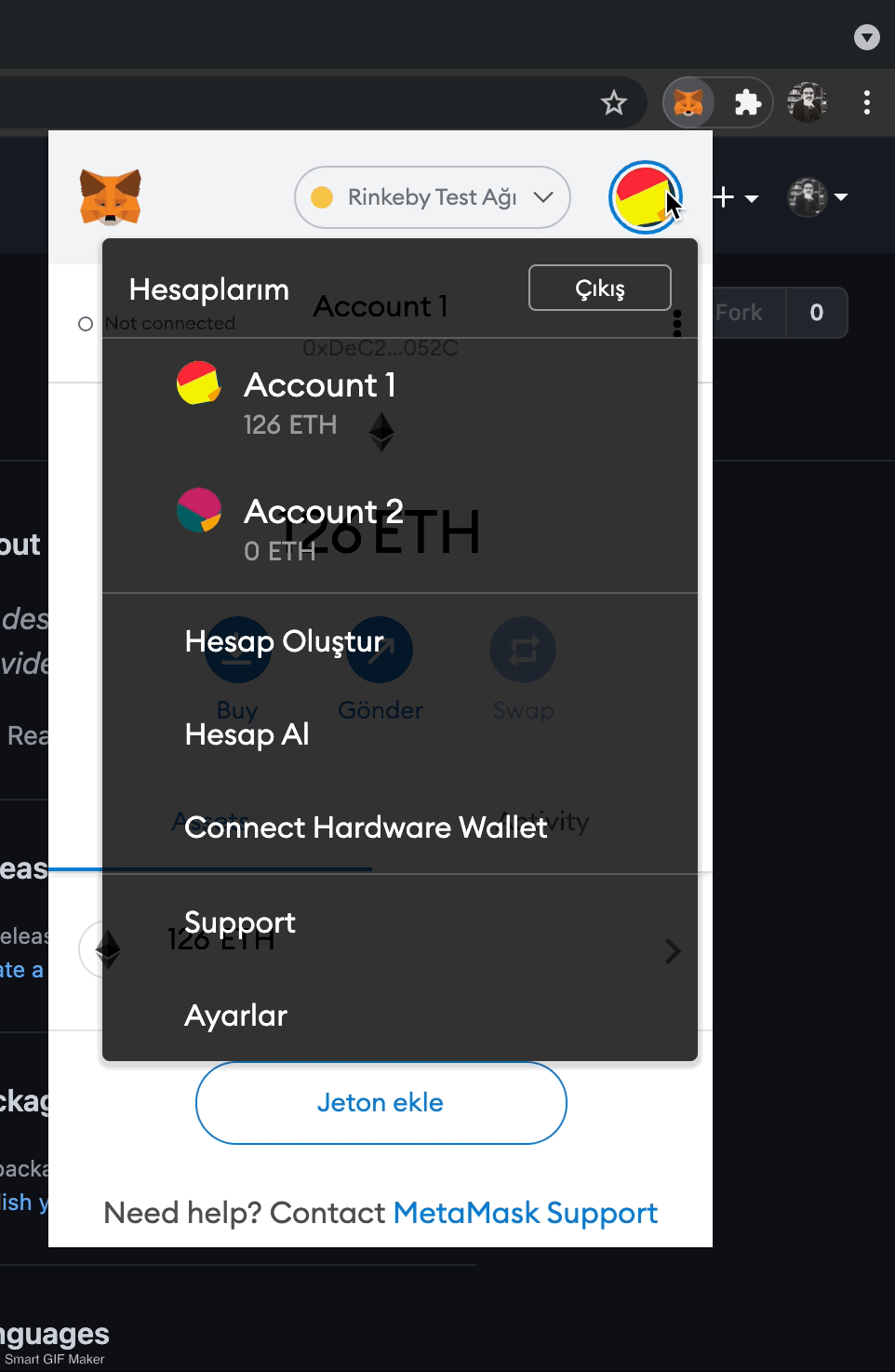
Now, we need to transfer some of our accounts in Ganache to MetaMask. We click on the key icon on the accounts section in Ganache and copy the Private Key.
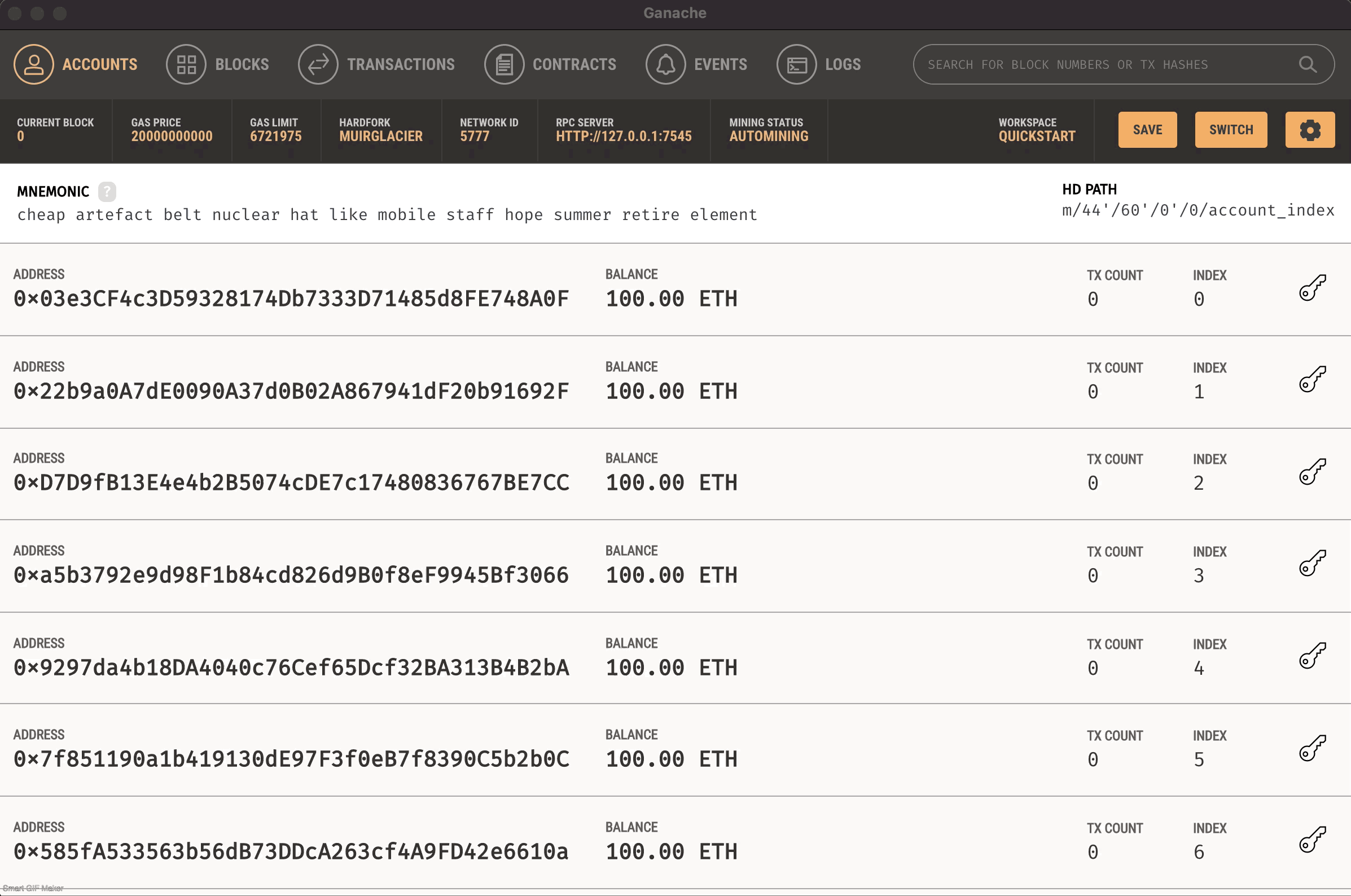
We go to the Import Account section in MetaMask and paste this private key.
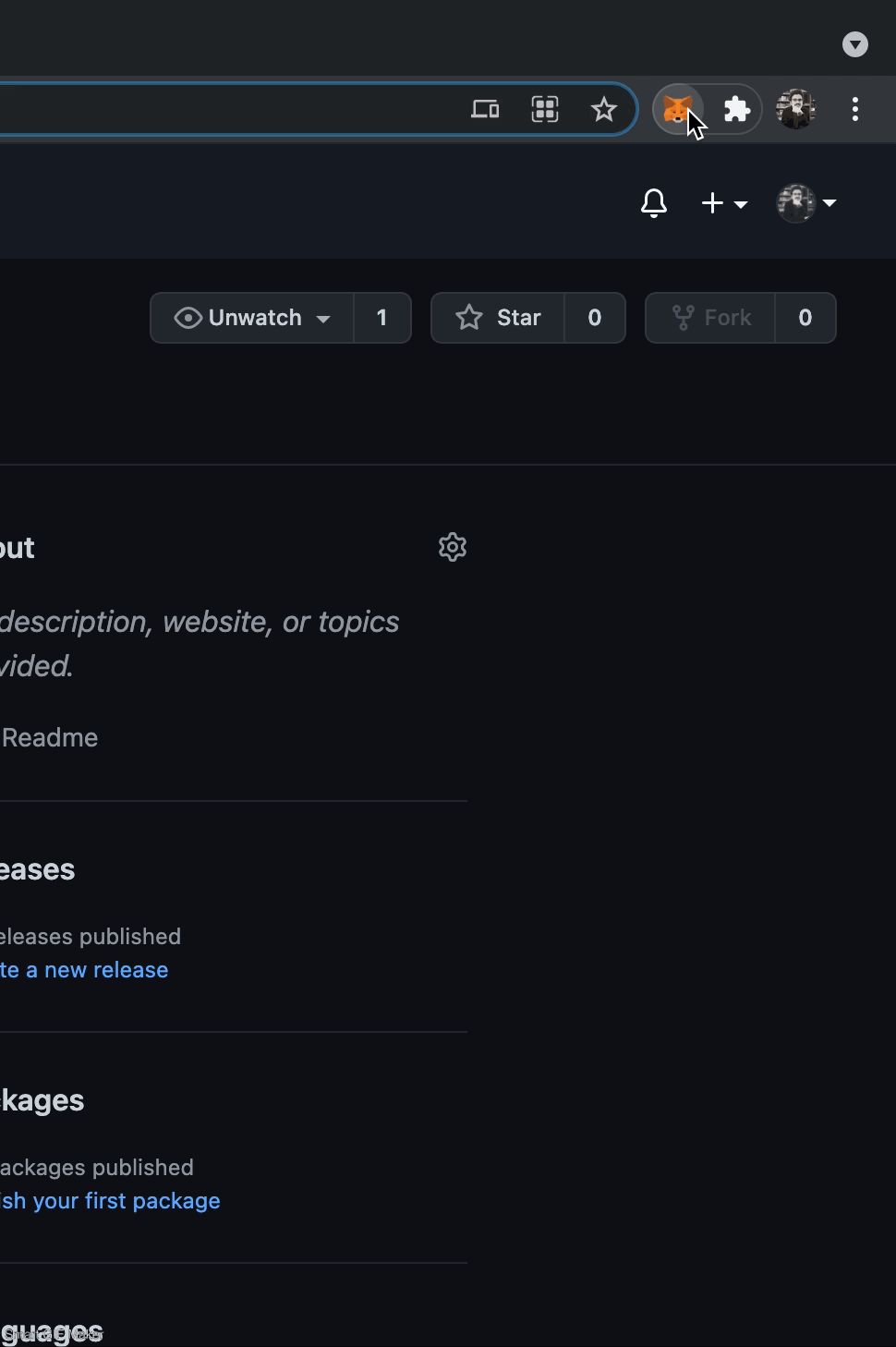
The first account is the owner of the contract according to our Contract. We have to import this account to MetaMask. Let’s import another account in order to carry out authentication tests.
Interaction with MetaMask and Preparing Web3.JS
Now we can code UI application. I created an application with VueJS. You can access the final version of the project from Github. I will share and explain the parts related to blockchain only. Because our goal here is not to learn VueJS.
We need to install MetaMask package in order to interact with MetaMask.
We need web3.js to make RPC call to a network in Ethereum. In the 3rd line, we can see that the package has been installed.
In the 9th line, we check whether MetaMask has been installed. In the 13th line, we listen to the event “accountsChanged” to get the new account address when the active account is changed in MetaMask.
In the 18th line, we send a request to MetaMask for connection. When this request is made, MetaMask will open and ask for some permissions to access the site. MetaMask will also ask for which accounts it can access to.
In the 20th line, we store the first account’s address. In the 30th line, we give MetaMask to web3.js as a provider. In this way, all transactions will be signed and sent to the network by MetaMask.
Preparing the Contract
Now we create an instance of our contract.
We pass the parameters, ABI and the contract address, in the 6th and 7th lines. We raised events like OrderPaid and OrderDelivered. You can subscribe and listen to events either collectively or individually.
Querying the Contract Balance
Let’s first query the contract balance.
In the 49th line, we query the contract balance from web3.js. The type of contract balance is WEI. In the 52nd line, we convert the WEI to ETH unit. Because in UI, we will show the contract balance in ETH.
Adding the Order
In the 45th line, we convert the order price from ETH to WEI. In the 46th line, we convert the delivery date to the uint data type. We convert the timestamp in JS to solidity type. We pass the current account of MetaMask to the send method.
Receiving Payment
We pass the total price of the order to the send method.
Delivering the Order
Payment Refunding
Order Fetching
In the 122nd line, we call the call function and get the order’s status. We convert the timestamp in solidity to JS type.
We have learned how to interact with UI. In the next post, we will send the contract to Rinkeby which is one of the test networks of Ethereum. We will also look at how to query the contract with API.
You can access the sample application from Github.
See you later.
Leave a comment
Reply to
Cancel reply